Debugging Decompiled EXE Code: A Complete Guide
As software developers and IT pros, we face the challenge of fixing issues in executable (EXE) files. These files are complex and hard to get into, especially without the source code. Luckily, decompiling EXE files can give us a peek into how the software works. This guide will teach you how to debug decompiled EXE code, giving you the tools and knowledge for effective troubleshooting.
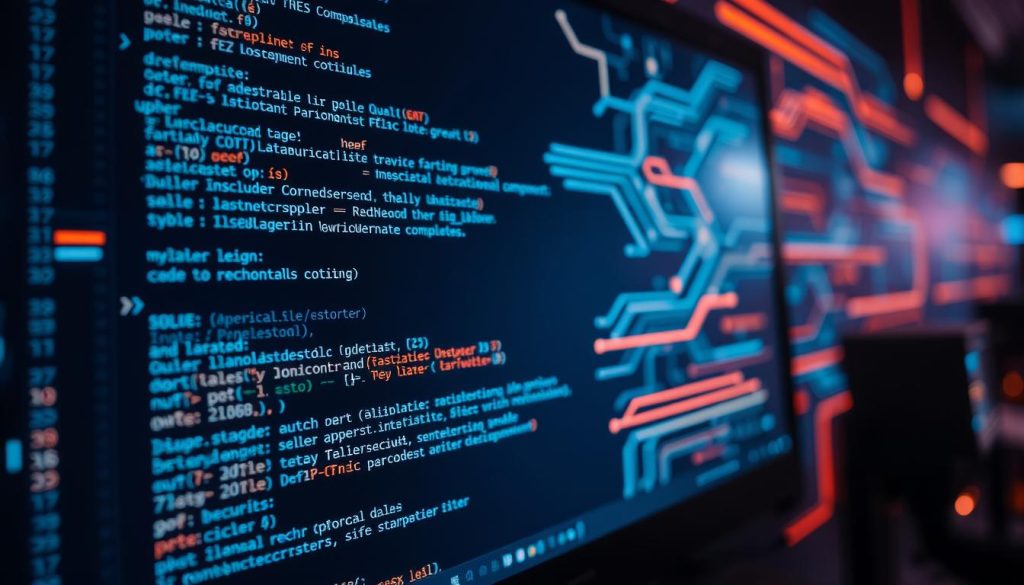
Key Takeaways
- Understand the fundamentals of EXE file structure and the purpose of decompilation
- Explore popular decompilation tools and software requirements for debugging
- Discover effective strategies for analyzing program flow and structure
- Leverage advanced debugging techniques to tackle complex issues
- Implement security considerations and best practices during the debugging process
Understanding the Basics of EXE Decompilation
To understand EXE decompilation, you need to know about EXE files and decompilation. This knowledge helps set up a debugging environment and use advanced techniques.
What is an EXE File?
An EXE file is a computer file that can be run directly by an operating system. It’s the final version of a program. EXE files have binary code that tells the computer how to run the software.
The Purpose of Decompilation
Decompilation turns an EXE file back into its original source code. It’s useful when you can’t find the original code or need to fix an app. By decompiling, developers can understand how the program works and fix it.
Common Decompilation Tools
- IDA Pro: A top tool for reverse-engineering, offering detailed info on the decompilation process and binary code.
- Ghidra: An NSA-developed tool for decompiling and analyzing various executable files.
- dnSpy: A .NET debugger and editor for decompiling .NET apps like Windows Forms and Console apps.
- dotPeek: A free tool from JetBrains for exploring .NET assemblies.
These tools are just a few examples. The right tool depends on your project’s needs and the type of executable files you’re working with.
“Decompilation is a valuable technique for understanding the inner workings of software, but it requires careful consideration of legal and ethical implications.”
Setting Up Your Debugging Environment
Creating reliable software needs a good debugging setup. This guide will help you set up a top-notch debugging environment. You’ll learn how to easily decompile and analyze your EXE files.
Equipping Your Toolkit
Start by gathering a set of debugging tools. These tools are key for decompiling. Some top choices include:
- IDA Pro
- OllyDbg
- Ghidra
- x64dbg
Get to know these tools’ features. This will help you pick the best one for your development environment and project.
Configuring Your System
Make sure your system is ready for decompilation and debugging. You might need to tweak software configuration settings. Also, ensure you have enough system resources and compatibility with your EXE file.
Hardware Requirement | Recommended Specification |
---|---|
Processor | Intel Core i5 or AMD Ryzen 5 (or higher) |
RAM | 8GB or more |
Storage | 500GB SSD or 1TB HDD |
Display | 1080p or higher resolution |
With a well-prepared debugging environment, you’ll be ready to handle EXE file decompilation and analysis.
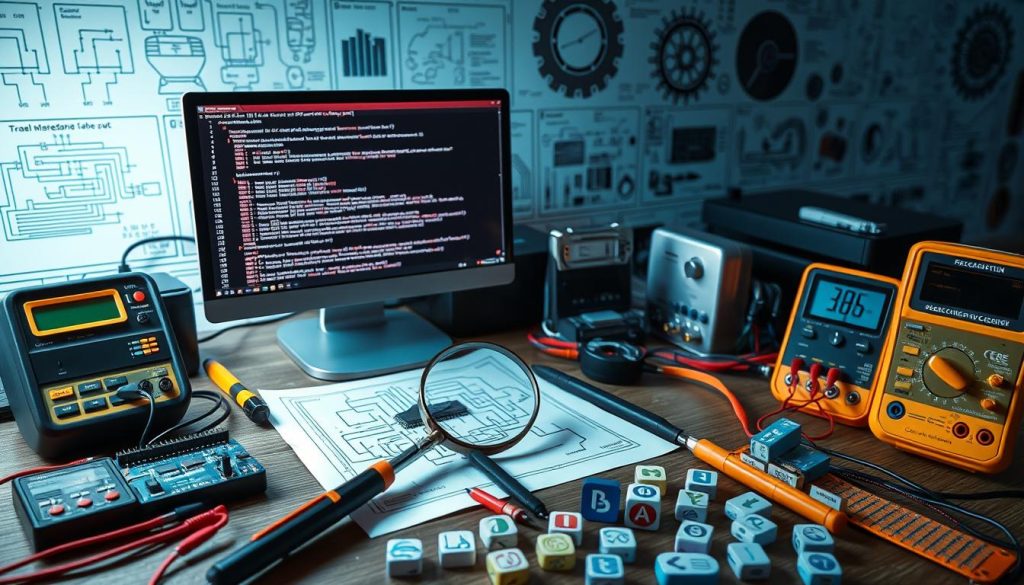
Essential Tools for Decompiling Executable Files
To unlock executable files, you need special tools. IDA Pro, Ghidra, OllyDbg, and x64dbg are key for decompiling EXE files. They help developers and analysts understand the code.
Popular Decompilers Overview
IDA Pro is a top choice for reverse engineering and malware analysis. It has features like code visualization and scripting. Ghidra, made by the NSA, is free and open-source. It has tools for binary analysis, like string and function identification.
OllyDbg is great for analyzing Windows binaries. x64dbg is for 64-bit Windows systems. Both are easy to use and have features for debugging and decompiling.
Debugging Software Requirements
- Robust decompiler software like IDA Pro, Ghidra, OllyDbg, or x64dbg
- Comprehensive debugging tools to analyze program execution and state
- Familiarity with assembly language and CPU architecture
- Knowledge of common software vulnerabilities and reversing techniques
Additional Support Tools
Tools like disassemblers, hex editors, and memory analyzers help a lot. They give deeper insights into executable files. This lets developers find hidden functions, spot vulnerabilities, and improve code.
“Reverse engineering is a powerful technique, but it should be used responsibly and ethically. Always consider the legal and ethical implications of your work.”
How to Debug Decompiled EXE Code for Software Troubleshooting
Debugging decompiled EXE code is key in fixing software problems. It lets you understand your app’s inner workings. This way, you can find and fix issues that pop up during use. We’ll look at different ways to debug decompiled code and make your software run better.
Systematic Code Analysis
Start by carefully looking over the decompiled code. Get to know the program’s layout, how it calls functions, and how data moves. Use debugging techniques like stepping through code, setting breakpoints, and checking variables. This helps you understand how the code works.
Error Identification and Resolution
While analyzing the code, watch for errors like syntax mistakes, runtime problems, or logical errors. Use problem-solving skills to find the main cause of these issues. You might need to change the code, fix external links, or tweak your development setup.
Leveraging Decompiler Capabilities
- Make the most of your decompilation tool‘s features to improve code analysis. Many tools offer advanced tools like cross-referencing, call graph views, and memory analysis.
- Use these tools to get a better grasp of the decompiled code. They can help you spot hidden connections or potential problems.
By using a methodical approach to debugging techniques and the power of decompilation tools, you can fix and improve your software. This ensures it works well and gives users a smooth experience.
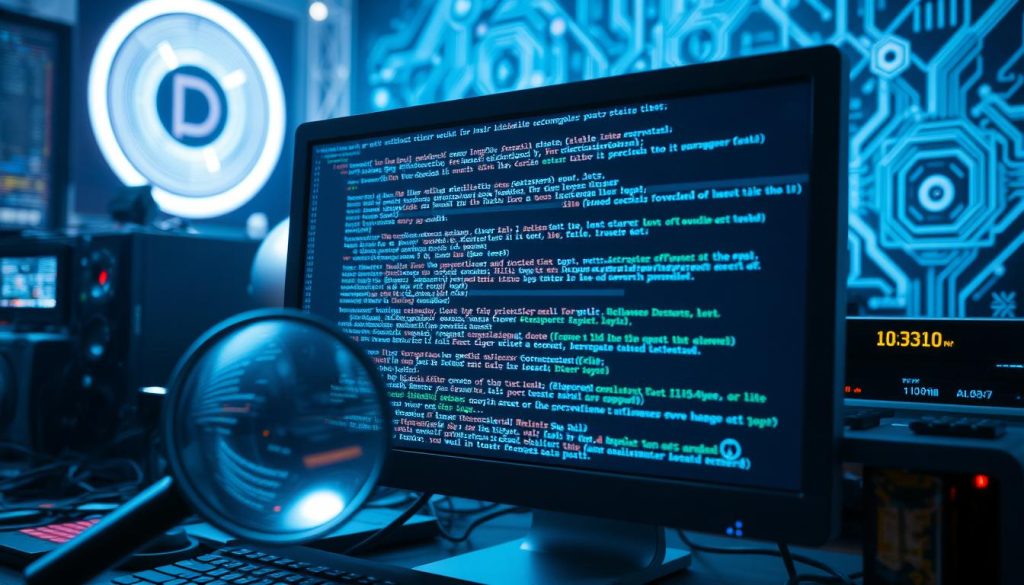
“Debugging is twice as hard as writing the code in the first place. Therefore, if you write the code as cleverly as possible, you are, by definition, not smart enough to debug it.” – Brian Kernighan
Common Challenges in EXE Decompilation
Decompiling EXE files is complex and often faces several obstacles. These hurdles can make it hard to get back the original source code. We’ll look at some common challenges developers and security analysts face with decompiled EXE code.
Code Obfuscation Issues
Code obfuscation is a big problem in EXE decompilation. Developers use tricks to make their code hard to understand and reverse-engineer. They rename variables and functions, mess with control flow, and add extra instructions. These code protection methods make it tough to get useful info from the decompiled code.
Missing Symbol Information
Decompilation struggles without symbol information. This includes function and variable names, and type details. Without this symbol table data, the code is hard to understand and analyze. It takes extra effort and research to figure out what the original programmer meant.
Anti-Debugging Techniques
Anti-reverse engineering techniques are another challenge. These are designed to stop decompilation and debugging. To get past these, you need special knowledge and tools. This adds more complexity to the decompilation process.
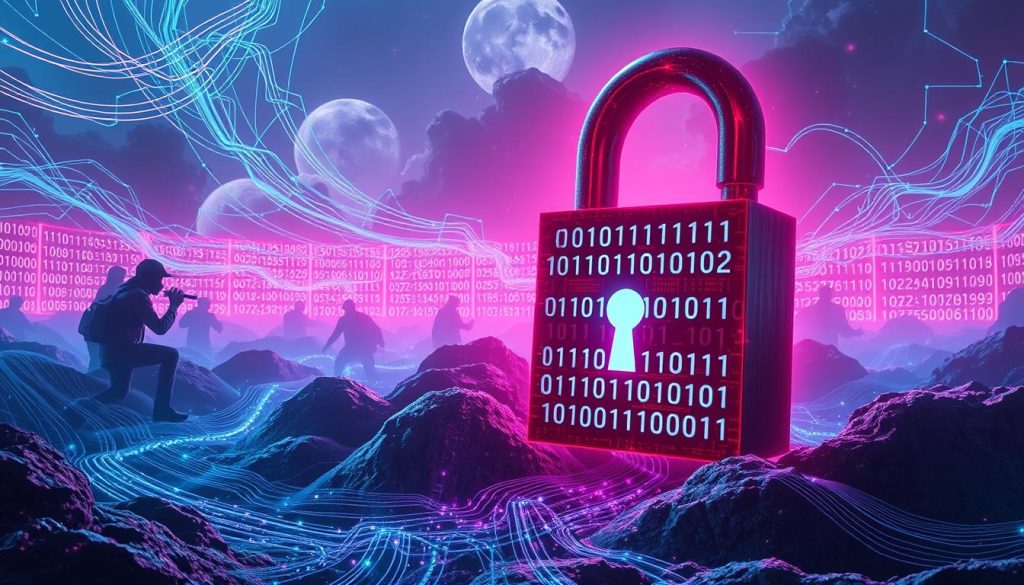
Beating these challenges needs technical skill, the right tools, and a deep understanding of decompilation and reverse engineering. By tackling these common issues, developers and security analysts can better analyze and debug decompiled EXE code. This helps ensure software security and integrity.
Analyzing Program Flow and Structure
When you work with decompiled executable (EXE) code, it’s key to understand the program’s flow and structure. This is vital for debugging and analysis. We’ll look at important methods for control flow analysis, function identification, and understanding the code structure of decompiled programs.
Tracing Control Flow
Tracing the control flow in decompiled code is a major task. It means finding the different paths, branches, and loops in the code. Knowing the control flow helps you see how the program works and where problems might be.
- Use control flow diagrams to see the program’s structure.
- Find conditional statements, loops, and jump instructions to follow the execution path.
- Look at the control flow to spot dead code, unreachable paths, and bugs.
Identifying Key Functions
Identifying key functions in decompiled code is also crucial. These functions are the heart of the program and help you understand its behavior.
- Spot common library functions and API calls in the code.
- Find the main entry and exit points of the program’s logic.
- Study function parameters, return values, and interactions to know their role.
Technique | Description | Benefits |
---|---|---|
Call Graph Analysis | Looks at the relationships and dependencies between functions in the code. | Gives a broad view of the program’s structure and points out key functions. |
Variable and Data Flow Tracking | Follows how variables and data are used and changed in the program. | Helps understand the program’s logic and finds data-related problems. |
Symbolic Execution | Studies the program’s behavior by using symbolic values instead of concrete ones. | Finds hidden paths and spots edge cases or vulnerabilities. |
By using these methods, you can deeply understand the control flow, function identification, and code structure of the decompiled executable. This knowledge lets you debug and analyze the program effectively.
Advanced Debugging Techniques
Exploring software troubleshooting, advanced debugging techniques are key. They help find complex issues. These methods offer deep insights into your decompiled EXE code.
Memory Analysis Methods
Memory analysis is a powerful tool. It lets you see the program’s memory state at runtime. By looking at memory dumps, you can find important info on variables and data structures.
This deep look into memory can change how you find and fix bugs.
Stack Trace Investigation
Stack trace analysis is also crucial. It shows the sequence of function calls before an error. By examining the call stack, you can find where errors start.
This helps you follow the code’s flow and find areas needing more attention.
Breakpoint Strategies
- Conditional breakpoints: These can only trigger under certain conditions. They help focus on specific code parts.
- Memory-based breakpoints: These pause execution when certain memory spots are accessed. They’re useful for tracking memory changes.
- Hardware breakpoints: These monitor CPU events. They offer deeper insights into program execution and hardware issues.
Learning these advanced debugging techniques improves your work with decompiled EXE code. You’ll find hidden bugs, boost performance, and create reliable software.
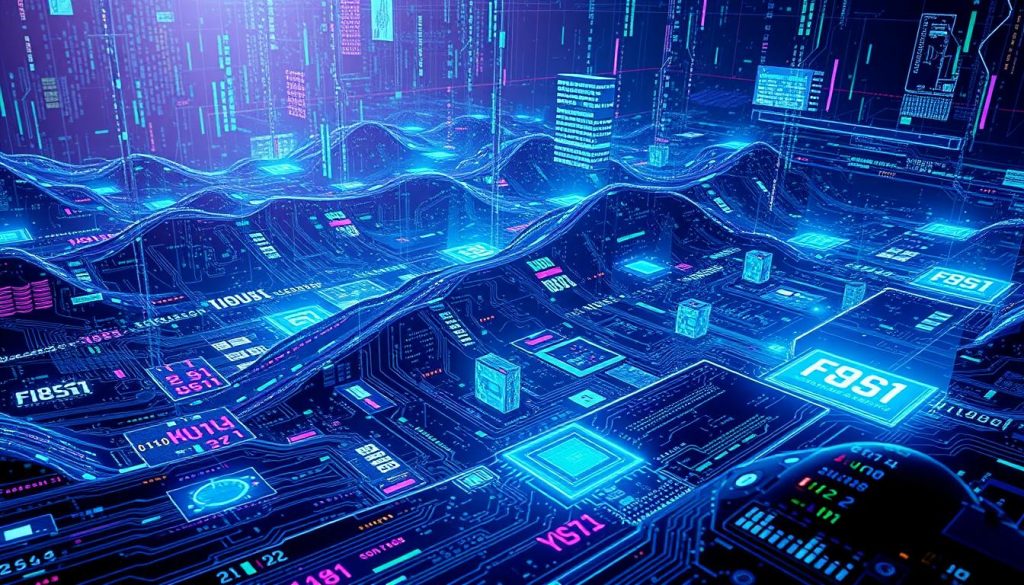
Handling Error Messages and Exceptions
Developers often face error messages and exceptions when working with decompiled EXE code. It’s important to handle these well for software troubleshooting. This helps find the root of the problem and guides the debugging process.
Exception handling is key when dealing with errors in decompiled code. It involves finding the cause of exceptions and fixing them. By understanding the exception and its context, developers can solve the issue and keep the app stable.
Error diagnosis is also vital in decompiling and debugging EXE files. Reading error messages and stack traces helps understand the program’s state. This knowledge helps developers find and fix the problems in the decompiled code.
Developers may also need to do crash analysis when apps crash or act strangely. Looking at crash dumps and memory snapshots helps figure out what went wrong. This lets developers fix the issues and prevent future crashes.
Learning to handle exceptions, diagnose errors, and analyze crashes helps developers work better with decompiled EXE code. It improves their ability to fix and enhance their apps’ performance.
Reverse Engineering Best Practices
When you start debugging decompiled executable (EXE) code, it’s key to follow best practices. These ensure your reverse engineering is both efficient and ethical. You’ll learn how to document your work well and analyze code effectively.
Documentation Methods
Good documentation is the base of any reverse engineering project. Start by writing down everything you find and think about the code. Keep a detailed log of your steps, tools used, challenges faced, and solutions found.
This log helps you understand your work better. It also makes it easier to share knowledge with others. This is useful for your team or future developers.
Code Analysis Strategies
For analyzing decompiled code, use a methodical and strategic approach. Get to know the program’s structure, including its main parts, data paths, and control systems. Use advanced techniques like control flow and data flow analysis.
This helps you find hidden links, spot vulnerabilities, and understand the software better. Also, think about the ethics of your work. Always respect the rights of the original creators and follow a strict code of ethics.
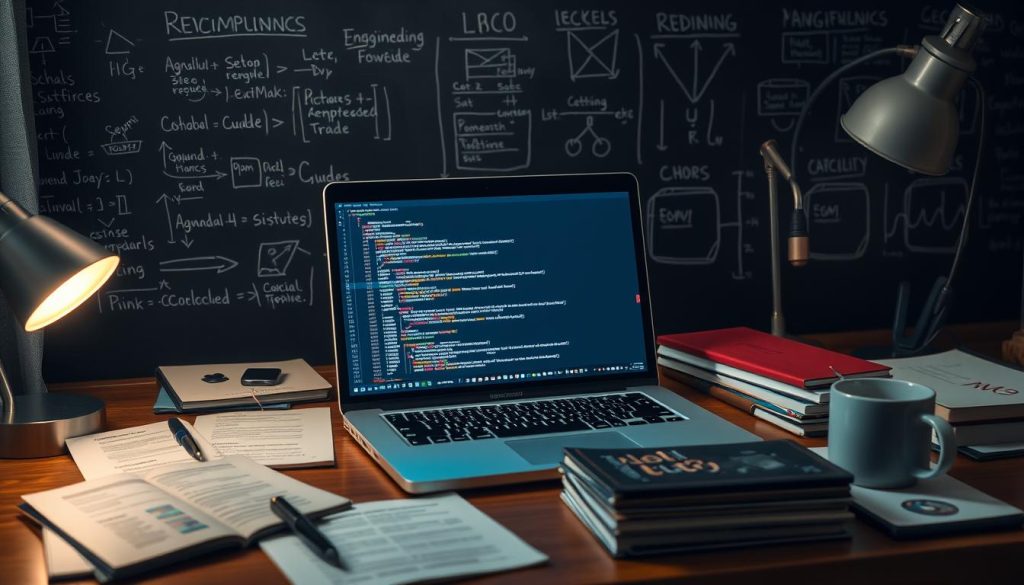
By following these best practices, you can fully explore decompiled EXE code. You’ll be able to fix software problems and help improve the apps you analyze.
Security Considerations During Debugging
As security experts, we must be very careful when debugging. Decompiling files can reveal sensitive data, risking our data protection. It’s vital to use secure debugging methods to protect important information.
When working with decompiled code, we need to protect data well. This means hiding or making hard to read sensitive info like passwords or API keys. Using secure debugging methods, like running in a safe, separate area, helps keep things secure.
Also, checking the code for vulnerabilities is key. We should look for things like buffer overflows or bad coding. This helps find and fix problems before they can be used by hackers.
By focusing on security in our debugging, we protect sensitive data. This reduces the chance of data breaches. It also makes sure the software is strong against threats.
Prior to debugging, learn how to extract strings and embedded resources from exe files.
Security Consideration | Recommended Approach |
---|---|
Data Protection | Mask or obfuscate sensitive data |
Secure Debugging | Utilize isolated, controlled environments |
Vulnerability Assessment | Scan decompiled code for security vulnerabilities |
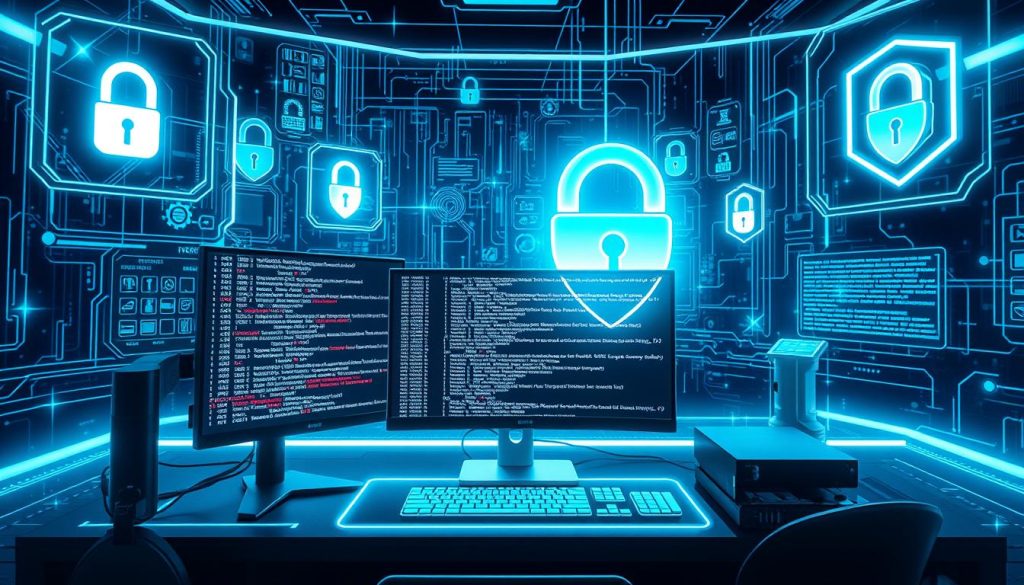
“Secure debugging is not just a best practice, it’s a necessity in today’s digital landscape. Overlooking these crucial security considerations can have dire consequences.”
Optimizing Decompiled Code Performance
Developers often face the challenge of optimizing decompiled executable (EXE) files. This is crucial for ensuring the software works well and provides a smooth user experience. We will look at strategies for finding performance bottlenecks and improving code efficiency.
Performance Bottleneck Identification
Finding the parts of the code that slow it down is the first step. This involves profiling the code and analyzing how long it takes to run. By using advanced tools, developers can find the code efficiency hotspots and understand the software’s performance.
Code Optimization Techniques
After finding the bottlenecks, the next step is to use optimization strategies to improve the software. This can include:
- Streamlining memory usage and reducing resource consumption
- Optimizing algorithm complexity and data structures
- Leveraging parallel processing and concurrency to improve performance tuning
- Implementing caching mechanisms to reduce redundant computations
- Eliminating unnecessary function calls and optimizing control flow
By carefully applying these techniques, developers can make the decompiled code run better. This ensures the software performs well and is responsive to users.
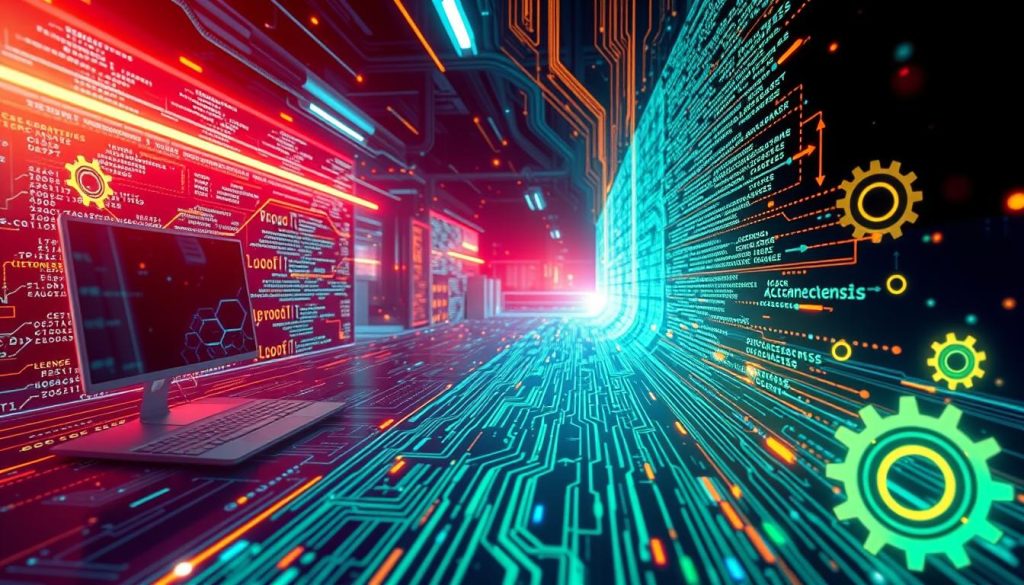
“Optimizing decompiled code performance is a critical step in ensuring the software’s functionality and user experience. By adopting a strategic approach to performance bottleneck identification and targeted optimization techniques, developers can unleash the full potential of their decompiled applications.”
Debugging Multi-threaded Applications
In today’s fast-paced software world, concurrent programming is key. But, multi-threaded apps bring their own set of problems. Issues like thread synchronization and race conditions can be tough to fix. This part will show you how to tackle these challenges.
Debugging multi-threaded apps often means dealing with race conditions. These happen when threads mess with shared resources in unpredictable ways. Tools like thread sanitizers and race detectors can help find and fix these problems.
Thread synchronization is also vital. It ensures threads work together smoothly. Learning about mutexes, semaphores, and condition variables can help you manage threads better and avoid deadlocks.
“Debugging multi-threaded applications requires a deep understanding of the underlying concurrency principles and the ability to think in terms of parallel execution.”
With the right techniques and tools, you can handle concurrent programming better. Remember, solving multi-threaded debugging problems needs sharp analytical skills, attention to detail, and a solid grasp of concurrency.
Integration with Source Control Systems
Debugging decompiled EXE code works best when it’s part of a source control system. Tools like Git and SVN help teams work together and manage versions. They make sure changes are tracked, shared, and can be easily found again.
Version Control Best Practices
It’s key to have good version control when dealing with decompiled code. Make sure to commit changes often, write clear commit messages, and use branches. This helps you go back to previous versions, find problems, and work well together.
Collaborative Debugging Approaches
Debugging decompiled EXE code is a team job. Using source control with debugging tools lets you review code live, share thoughts, and learn from each other. This way, everyone can help figure out and fix problems, making the app more stable and reliable.
FAQ
What is an EXE file?
An EXE file is a computer file that runs programs or applications. It can be executed directly on a computer.
What is the purpose of decompilation?
Decompilation turns an EXE file back into source code. This makes it easier to analyze, understand, or modify the original program.
What are some common decompilation tools?
Tools like IDA Pro, Ghidra, OllyDbg, and x64dbg help analyze decompiled code. They show the structure and function of the code.
How do I set up an effective debugging environment?
First, install necessary software and check your hardware. Then, optimize your system settings for better decompilation and analysis.
What are some common challenges in EXE decompilation?
Challenges include code obfuscation, missing symbols, and anti-debugging techniques. These make decompilation harder.
How can I analyze the program flow and structure of decompiled code?
To understand the code, analyze control flow, identify functions, and map the code structure. This helps grasp the program’s flow.
What are some advanced debugging techniques?
Advanced techniques include memory analysis, stack trace investigation, and using breakpoints. These help find complex issues.
How do I handle error messages and exceptions in decompiled code?
To handle errors, interpret diagnostic info, find the cause, and use troubleshooting strategies. This fixes issues.
What are some best practices for reverse engineering?
Best practices include documenting thoroughly, analyzing code strategically, and keeping ethical standards. These ensure quality and integrity.
How can I ensure security when debugging decompiled code?
To stay secure, protect sensitive data, check for vulnerabilities, and follow secure debugging practices. This keeps your work safe.
How can I optimize the performance of decompiled code?
Improve performance by finding bottlenecks and using optimization techniques. This makes the code run better.
How do I debug multi-threaded applications?
Debugging multi-threaded apps requires strategies for thread synchronization and race conditions. This ensures smooth operation.
How can I integrate debugging with source control systems?
Integrate debugging with source control by following version control best practices. This helps in team environments.
Need help decompiling your file? Our exe decompiler online guarantees success.